Deep Dive into React Server Components

Steven Rugg
@Steven Rugg
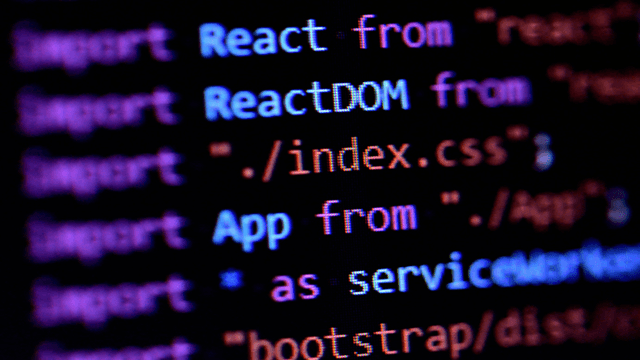
React Server Components (RSCs) represent a significant shift in how React applications handle rendering, enabling developers to build more efficient and scalable applications. Introduced as part of the React 18 release, Server Components allow developers to blend the power of server-side rendering (SSR) with client-side interactivity, leading to faster load times and reduced bundle sizes.
In this article, we'll explore what React Server Components are, how they differ from traditional React components, and how you can use them to improve the performance of your web applications.
1. What are React Server Components?
React Server Components are a new type of React component that run exclusively on the server. Unlike traditional React components, which run on the client, Server Components enable you to offload heavy computation and data-fetching to the server without sacrificing interactivity on the client side. This approach reduces the amount of JavaScript sent to the browser, leading to faster initial load times and improved user experiences.
Key Characteristics:
- Server-Side Execution: React Server Components execute on the server and send lightweight HTML or component instructions to the client.
- No Client-Side JavaScript: These components are never sent to the browser, reducing the amount of JavaScript the client needs to load and execute.
- Streaming Capabilities: Server Components can stream data as it becomes available, speeding up the delivery of content to users.
2. How Do React Server Components Work?
React Server Components work by separating the concerns of rendering and interactivity between the server and the client. Here’s how they function:
- Server Components Rendering: When a Server Component is requested, it is rendered on the server. The server processes all the logic, such as data fetching and heavy computations, and generates the necessary HTML.
- Streaming to the Client: Instead of waiting for the entire component to be rendered before sending the data, React streams the component in chunks to the client as the data becomes available.
- Client-Side Integration: Once the server has streamed the rendered content, React hydrates the client-side components, adding interactivity where needed.
By utilizing this hybrid approach, developers can ensure that the server handles the heavy lifting while the client handles only the essential JavaScript for interactivity.
3. Key Benefits of React Server Components
a. Reduced Client Bundle Size
One of the primary benefits of React Server Components is the reduction in client-side bundle size. Since Server Components run on the server and don't include JavaScript for the client, they drastically cut down the amount of JavaScript sent to the browser. This leads to faster load times, especially for complex applications.
b. Improved Performance
React Server Components enable server-side data fetching and processing, which can significantly improve performance. Instead of loading data on the client, Server Components fetch and render data on the server, streaming the content to the user as soon as it's ready. This makes it possible to serve dynamic content faster without waiting for client-side API calls.
c. Simplified Data Fetching
With Server Components, data fetching can be simplified. Instead of managing API requests on the client, you can fetch data on the server during the rendering process. This removes the need for complex client-side state management and reduces the likelihood of issues like "waterfall" network requests.
4. Comparison with Traditional Client-Side Components
To understand how React Server Components differ from traditional React components, let's look at a side-by-side comparison:
Feature | Traditional Client-Side Components | React Server Components |
---|---|---|
Rendering Location | Client | Server |
Interactivity | Fully interactive | Mostly static; client hydration for interactivity |
Data Fetching | Handled on the client | Handled on the server |
Bundle Size | Larger (JavaScript sent to the client) | Smaller (no JS sent to client) |
Performance | Depends on client resources | Optimized through server-side rendering and streaming |
Server Components excel in scenarios where performance and initial load time are critical, while client-side components remain essential for building highly interactive features.
5. Implementing React Server Components: A Step-by-Step Guide
Let’s walk through the process of implementing React Server Components in a sample project.
Step 1: Set Up Your React App
To get started, ensure that your React project is set up with React 18. If you’re starting from scratch, use the following command to create a new React application:
npx create-react-app my-app --template cra-template-rsc
This will create a new React app pre-configured with Server Components support.
Step 2: Define a Server Component
Next, create a Server Component in your application. Server Components are simple to define, just like traditional React components, but they only run on the server. Here’s an example:
// src/components/MyServerComponent.server.js
import React from 'react';
const MyServerComponent = () => {
'use server'
const data = fetchSomeDataFromAPI(); // Fetch data on the server
return (
<div>
<h1>Data from the server: {data}</h1>
</div>
);
};
export default MyServerComponent;
Notice the 'use server'
directive, and the naming convention **/*.server.js which indicate that this component will only run on the server.
Step 3: Use the Server Component in Your App
To use the Server Component in your app, you can render it within a client component or directly in your server-side rendering function:
// src/App.js
import React from 'react';
import MyServerComponent from './components/MyServerComponent';
const App = () => {
return (
<div>
<MyServerComponent />
</div>
);
};
export default App;
Step 4: Run the Application
Now, start the React development server using:
npm start
OR if you are using Next.js
npx next dev
When you load the app, React will render the MyServerComponent on the server and send the pre-rendered HTML to the browser, reducing the amount of JavaScript that needs to be loaded on the client.
6. Use Cases for React Server Components
React Server Components are best suited for scenarios where performance and server-side processing are essential. Here are some common use cases:
- Content-heavy applications: Blogs, news websites, or any application where a lot of content needs to be displayed dynamically can benefit from Server Components.
- E-commerce platforms: Product catalogs that require fast page loads can leverage Server Components for data fetching and rendering on the server.
- Enterprise applications: Applications with large datasets that need to be displayed efficiently can use Server Components to handle data processing on the server side.
Conclusion
React Server Components mark a new era in web development by bridging the gap between server-side rendering and client-side interactivity. By reducing the client-side bundle size and allowing for server-side data fetching, Server Components offer significant performance improvements, especially for content-rich applications. As more developers adopt this new feature in React 18, the future of web performance and scalability looks brighter than ever.
Stay tuned for more updates as the React ecosystem continues to evolve!